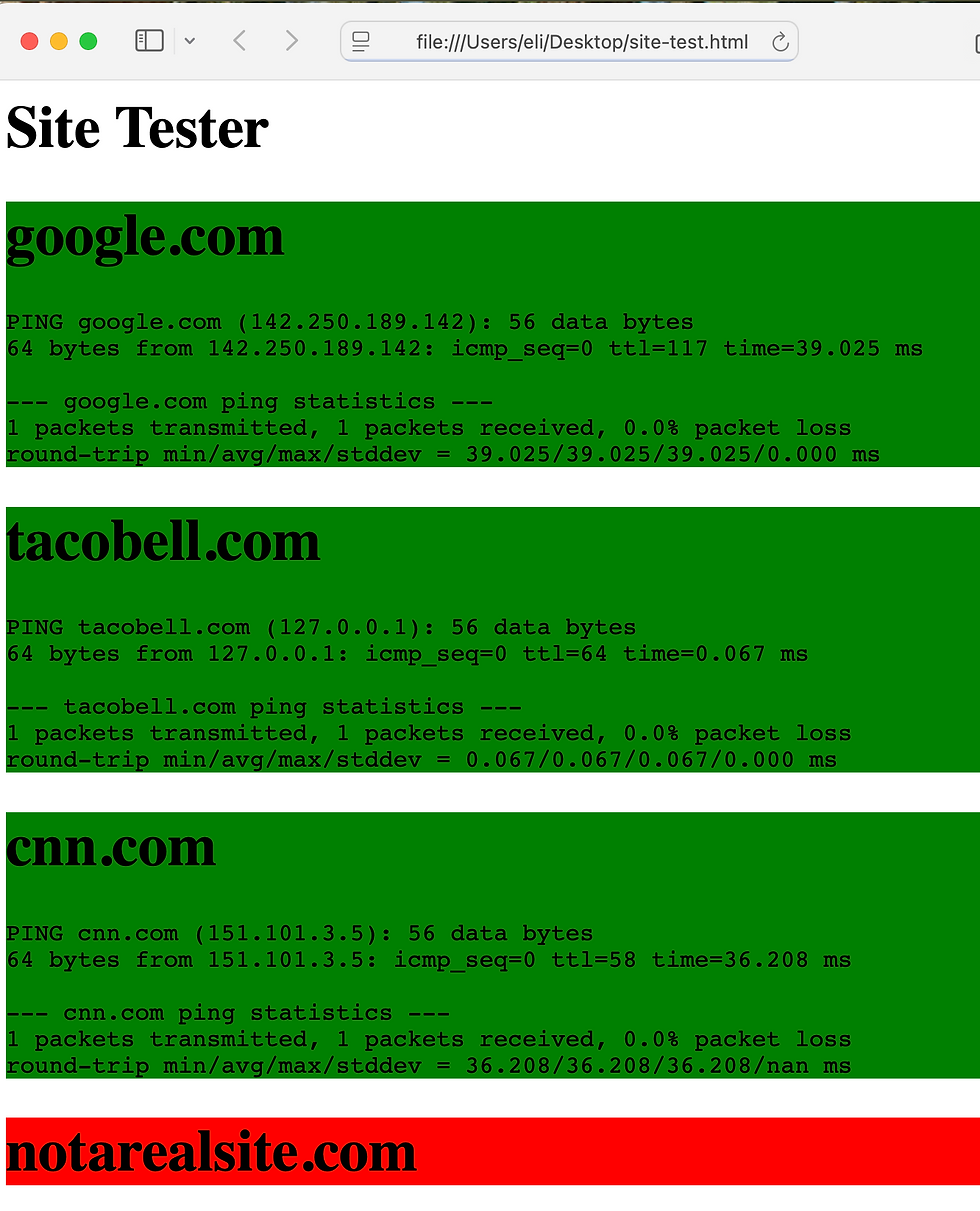
System: This project was created on MacOS. Since it interacts with the operating system if you use it on another OS you may need to modify it.
Note: For Ubuntu check for '1 packet received' in if statement instead of the plural 'packets'.
Note: This project won't work for Windows
This lab shows you how to use the OS module in Python to ping hosts and then based on the response dynamically write an auto updating HTML dashboard.
You import the OS module and the sleep function from the time module.
We then create a list of hosts to ping. These can be DNS names or IP Addresses.
We start a while True loop so that we can continously process responses and update an HTML dashboard.
We start the page variable value with the meta tag to force the page to auto-refresh every 5 seconds, and add a title for the App page in H1 tags.
We then loop through each value in the host_list list.
We use the popen os function to send the ping command to the operating system, and then use the read() method to take the response as a text avlue and send that value to the variable called response. The ping command uses an f string to add the host name to the command. We use -c 1 to only ping once.
We then use an if else statement to determine if the ping command worked. Depending on the result we set the variable value for color to either green or red.
We then use += to add to the page variable a div with a background color that was determined, the name of the host pinged, and then the full response.
Then we write the full value of page to site-test.html.
Finally we pause the code for 2 seconds.
import os
from time import sleep
host_list = ['google.com',
'tacobell.com',
'cnn.com',
'notarealsite.com']
while True:
page = '''<meta http-equiv="refresh" content="5">
<h1>Site Tester</h1>'''
for host in host_list:
response = os.popen(f'ping -c 1 {host}').read()
print(response)
if '1 packets received' in response:
color = 'green'
else:
color = 'red'
page += f'''<div style="background-color:{color};">
<h1>{host}</h1>
<pre>{response}</pre>
</div>'''
with open('site-test.html', 'w') as file:
file.write(page)
sleep(2)
Comments