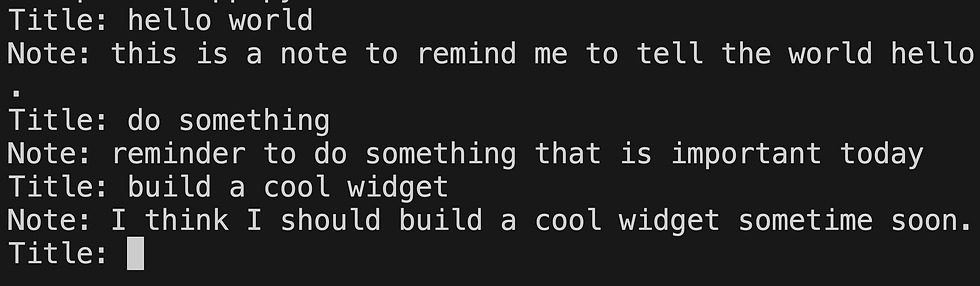
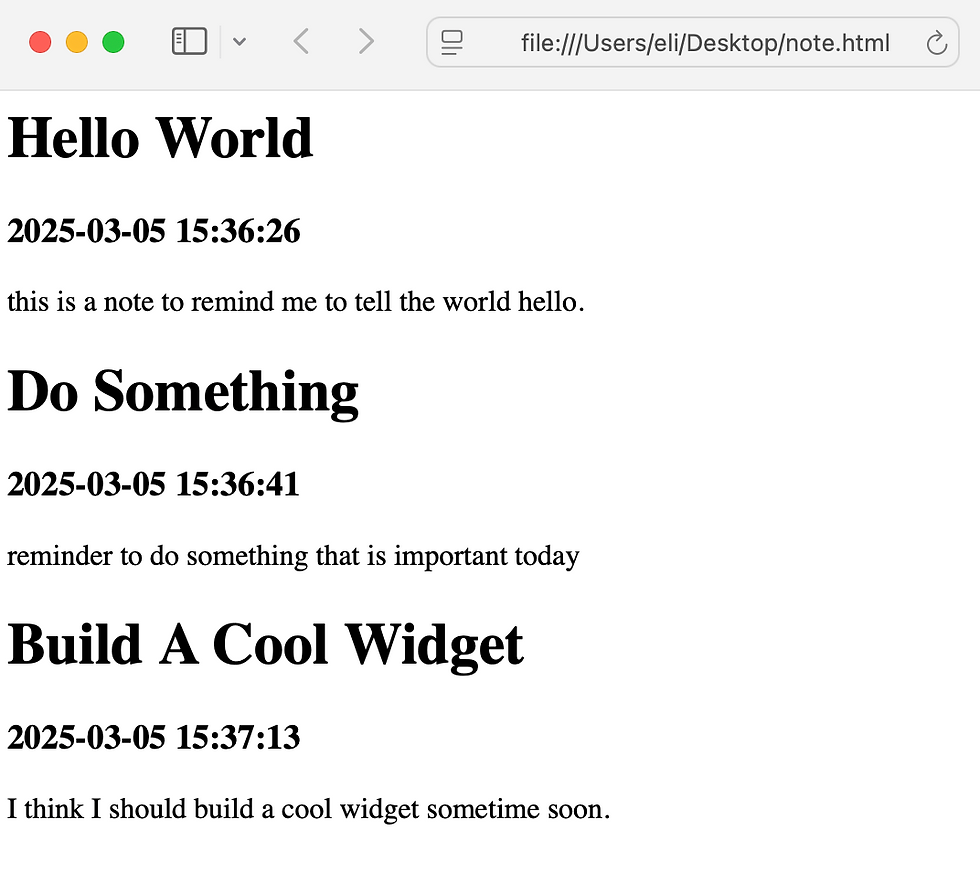
This is a simple app that allows you to take notes from the command line and they will be saved into an HTML page.
The app will ask you for a Title, a Note and will create a Timestamp. It will then append these to an HTML page that you can open with a web browser.
We use the datetime.now() function from the datetime module to get the current timestamp and then we format that timestamp using strftime().
The script runs in a while True loop so that you can keep adding notes. We use the with open() function to write to the HTML page with an f string. We use the title() method to capitalize the first letter of each word in the Title.
from datetime import datetime
while True:
title = input('Title: ')
note = input('Note: ')
timestamp = datetime.now().strftime('%Y-%m-%d %H:%M:%S')
with open('note.html', 'a') as file:
file.write(f'''<h1>{title.title()}</h1>
<h3>{timestamp}</h3>
<p>{note}</p>''')
Comments